Native App Print for Windows
Overview
Sample programs to test the B-gate and peripheral devices connected to the B-gate.
The sample programs are developed in C ++ (MFC) and C #.
Visual Studio 2008 or later is recommended.
To test the sample, It is important to select the correct connection interface for the communication between the sample and the B-gate.
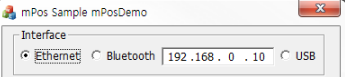
When connecting via Ethernet or Wi-Fi, select Ethernet and enter the IP address of the B-gate.
When connecting via Bluetooth, select Bluetooth and enter the COM port number paired with host.
There is no need to enter a value in the text box when connecting via USB.
Caution |
Refer to the 'User Manual' for how to check the assigned IP address of the B-gate or MAC address of Bluetooth. |
Below is the class name of each device.
Device types |
Class Name |
Label Printer |
CMposControllerLabel |
POS Printer |
CMposControllerPrinter(B-gate all-in-one printer included) |
HID |
CMposControllerHID |
MSR |
CMposControllerMSR |
Barcode Scanner |
CMposControllerScanner |
RFID |
CMposControllerRFID |
Dallas Key |
CMposControllerDallasKey |
NFC |
CMposControllerNFC |
Customer Display |
CMposControllerBCD |
USB-to-Serial |
CMposControllerTTYUSB |
Scale |
CMposControllerScale |
Sample App Download
Sample App Download for WindowsSample Code
SDK Download for WindowsOverview
In order to use a sample, the appropriate Interface configurations will be needed in B-gate where each sample program will connect to.
When connecting via Ethernet or WiFi, select Ethernet and then enter the IP address of the B-gate.
When connecting via Bluetooth. Select Bluetooth and then enter the COM port number which is paired with the host.
When connecting via USB, you do not need to enter a value in the text box.
Caution |
Please refer to the “User Manual” for details on how to check the B-gate’s assigned IP address or the Mac address of the Bluetooth. |
Below are the Class names of each device.
Device types |
Class name |
Label Printer |
CMposControllerLabel |
POS Printer |
CMposControllerPrinter (including B-gate solid-type printer) |
HID |
CMposControllerHID |
MSR |
CMposControllerMSR |
Barcode Scanner |
CMposControllerScanner |
RFID |
CMposControllerRFID |
Dallas Key |
CMposControllerDallasKey |
NFC |
CMposControllerNFC |
Customer Display |
CMposControllerBCD |
USB-to-Serial |
CMposControllerTTYUSB |
Scale |
CMposControllerScale |
1 Preparing to Use B-gate Device
An initializing process is needed to use a B-gate device.
This is a sample of a Printer device.
CMposControllerPrinter* pMPosPrinter = new CMposControllerPrinter(); pMPosPrinter->selectInterface(m_nInterface, m_strPort); //pMPosPrinter->selectCommandMode(MPOS_COMMAND_MODE_BYPASS); //When connecting directly to device //pMPosPrinter->setTimeout(5);//openService Timeout configuration long lResult = pMPosPrinter->openService(); if(pMPosPrinter->openService() != MPOS_SUCCESS) { //open fail } //open success |
* The m_nInterface value in the Select Interface function is the value of the Interface of the B-gate that is to be connected, while m_strPort is the actual address value.
1) If the IP address of B-gate to be connected with Ethernet or Wi-Fi is ‘192.168.0.10’
pMPosPrinter->selectInterface(MPOS_INTERFACE_ETHERNET, _T("192.168.0.10")); |
2) If the COM port number of B-gate that is connected with Bluetooth and paired with a host is ‘COM3’
pMPosPrinter->selectInterface(MPOS_INTERFACE_BLUETOOTH, _T("COM3")); |
3) If connecting with a USB
pMPosPrinter->selectInterface(MPOS_INTERFACE_USB, _T("")); |
* When connecting a device, the selectCommandMode function is used to select whether to connect directly or through the B-gate. This can be left out if connecting through B-gate. When calling the function, you can use ‘MPOS_COMMAND_MODE_DEFAULT’.
If connecting a device directly, you must call ‘MPOS_COMMAND_MODE_BYPASS’.
pMPosPrinter->selectCommandMode(MPOS_COMMAND_MODE_BYPASS); |
Please refer to the B-gate SDK manual for devices that can be connected directly and not through B-gate.
* The setTimeout function is to configure the time limit on how long openService runs. If the function is not used, the default value is 3 seconds. When using, the value unit is in ‘seconds (sec)’.
pMPosPrinter->setTimeout(5); //When configuring timeout as 5 seconds |
* The openService function forms an actual connection with a device, checks whether or not the device can be used, and then returns a result value.
pMPosPrinter->openService(); |
If 2 or more of the same type of device is used, the openServiceById() function can be used. The following example initializes an outside printer connected to the B-gate.
pMPosPrinter->openServiceById(11) |
2 Ending Use of B-gate Device
To finish and close the B-gate device. Please refer to the example below.
pMPosPrinter->closeService(0); |
For the closeService function’s parameters, you can enter the appropriate time required,
if more time is needed to send the remaining data in the transmission buffer when closing a
device. The value unit is in ‘seconds (sec)’.
Print Sample
This sample shows how to use a printing device. The sample is made of Print, Check Status, and Pagemode.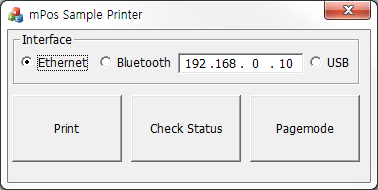
Print Sample prints and cuts texts/barcodes/image files.
//initilize mpos. … pPrinter->printText(_T("mPosWindows SDK Sample\r\n"), &fontattr); pPrinter->print1DBarcode(_T("1234567890123456"), MPOS_BARCODE_CODE128, 100, 3, MPOS_ALIGNMENT_CENTER, MPOS_BARCODE_TEXTBELOW); pPrinter->printBitmapFile(_T("Logo.bmp"), MPOS_IMAGE_WIDTH_ASIS, MPOS_ALIGNMENT_CENTER, 0, FALSE, FALSE); pPrinter->cutPaper(MPOS_PRINTER_FEEDCUT); //finalize mpos. |
Texts are printed using the printText while text attributes can be configured with FontAttribute.Please refer to item 5-3-4-2 Printer Sample - 2 FontAttribute for examples of FontAttribute.
Please refer to item 5-3-4-2 Printer Sample - 3 ESC Sequence for examples of ESC Sequence.
Barcode prints the Code128 barcode. To print other types of barcodes, change the second parameter of the print1DBarcode function, which is the symbology value.
Please refer to the B-gate SDK manual for the constant values defined according to each type.
To change the width of a barcode, change the 4th parameter, which is the barWidth value.
The path of the image ‘Logo.bmp’ file in the example is configured as the relative path.
The image width is the value that prints the image at the same size as the original image file.To change the image width before printing, enter the desired value in the second parameter.
2 FontAttribute
Font attributes, that can be configured using the FontAttribute are font type, font size(scale unit), bold, underline, reverse and align. When encoding additional character strings, values being used can be configured.
1) Configure ‘font type’ using FontAttribute in the printText function (default value: Font A)
FONTATTRIBUTE fontTypeStruct = {0,}; fontTypeStruct.fontType = MPOS_FONT_TYPE_B; //set ‘Font B’ pPrinter->printText(_T("mPosWindows SDK Sample\r\n"), &fontTypeStruct); |
2) Configure ‘font size’ using FontAttribute in the printText function (default value: width times 1, height times 1)
FONTATTRIBUTE fontTypeStruct = {0,}; fontTypeStruct.fontSize = MPOS_FONT_SIZE_WIDTH_1 | MPOS_FONT_SIZE_HEIGHT_1;//width times 2, height times 2 pPrinter->printText(_T("mPosWindows SDK Sample\r\n"), &fontTypeStruct); |
3) Configure ‘bold’ using FontAttribute in the printText function (default value: normal)
FONTATTRIBUTE fontTypeStruct = {0,}; fontTypeStruct.bold = TRUE; //set ‘bold property’ pPrinter->printText(_T("mPosWindows SDK Sample\r\n"), &fontTypeStruct); |
4) Configure ‘underline’ using FontAttribute in the printText function (default value: no underline)
FONTATTRIBUTE fontTypeStruct = {0,}; fontTypeStruct.underline = TRUE; //set ‘underline property’ pPrinter->printText(_T("mPosWindows SDK Sample\r\n"), &fontTypeStruct); |
5) Configure ‘reverse’ using FontAttribute in the printText function (default value: normal)
FONTATTRIBUTE fontTypeStruct = {0,}; fontTypeStruct.reverse = TRUE; //set ‘reverse property’ pPrinter->printText(_T("mPosWindows SDK Sample\r\n"), &fontTypeStruct); |
6) Configure ‘reverse’ using FontAttribute in the printText function (default value: normal)
FONTATTRIBUTE fontTypeStruct = {0,}; fontTypeStruct.alignment = MPOS_ALIGNMENT_CENTER; //set ‘alignment property’ pPrinter->printText(_T("mPosWindows SDK Sample\r\n"), &fontTypeStruct); |
7) Configure ‘textEncoding’ value using FontAttribute in the printText function Configure the sample to Korean. The language value of the user’s OS will be the default value.
FONTATTRIBUTE fontTypeStruct = {0,}; fontTypeStruct.textEncoding = 949;//set korean encoding value. pPrinter->printText(_T("mPosWindows SDK Sample\r\n"), &fontTypeStruct); |
3 ESC Sequence
Font attributes that can be configured using ESC Sequence are font type, font size(scale unit), bold, underline, reverse, and align. Attributes configured with ESC Sequence will apply only to character strings included when the function is called. Once the function call ends, the attributes will automatically initialize. The ‘textEncoding’ attribute of the FontAttribute is not supported in ESC Sequence. To change the character string encoding value, the setTextEncoding function must be used.
The ESC variable used in the sample is “\x1B” value.
A blank space is added to the front of the character string in order to distinguish ESC Sequence commands.
1) Configure ‘font type’ attribute using ESC Sequence in the printText function
ESC Seq: ESC + “|0fT” (Font A, default value), ESC + “|1fT” (Font B),
ESC + “|2fT” (Font C)
pPrinter->printText(ESC + _T("|1fT mPosWindows SDK Sample\r\n"), null); |
2) Configure ‘font size’ attribute using ESC Sequence in the printText function
ESC Seq: ESC + “|1C” (width times 1, default value), ESC + “|2C” (width times 2),
ESC + “|3C” (height times 2), ESC + “|4C” (width times 2, height times 2)
pPrinter->printText(ESC + _T("|4C mPosWindows SDK Sample\r\n"), null); |
3) Configure ‘bold’ attribute using ESC Sequence in the printText function
ESC Seq: ESC + “|bC” (configure), ESC + “|!bC” (cancel, default value)
pPrinter->printText(ESC + _T("|bC mPosWindows SDK Sample\r\n"), null); |
4) Configure ‘underline’ attribute using ESC Sequence in the printText function
ESC Seq: ESC + “|uC” (configure), ESC + “|!uC” (cancel, default value)
pPrinter->printText(ESC + _T("|uC mPosWindows SDK Sample\r\n"), null); |
5) Configure ‘reverse’ attribute using ESC Sequence in the printText function
ESC Seq: ESC + “|rvC” (configure), ESC + “|!rvC” (cancel, default value)
pPrinter->printText(ESC + _T("|rvC mPosWindows SDK Sample\r\n"), null); |
6) Align using ESC Sequence in the printText function
ESC Seq: ESC + “|lA” (align left, default value), ESC + “|cA” (align at center),
ESC + “|rA” (align right)
pPrinter->printText(ESC + _T("|cA mPosWindows SDK Sample\r\n"), null); |
These are additional descriptions of configurable attributes in ESC Sequence.
7) Cut using ESC Sequence in the printText function
ESC Seq: ESC + “|90P” (cut in place), ESC + “|90fP” (cut after feeding)
pPrinter->printText(ESC + _T("|90fP mPosWindows SDK Sample\r\n"), null); |
8) Feed using ESC Sequence in the printText function
ESC Seq: ESC + “|5lF” (5 line feeding, line unit feeding as much as number)
pPrinter->printText(ESC + _T("|5lF mPosWindows SDK Sample\r\n"), null); |
9) Initialize attributes using ESC Sequence in the printText function
ESC Seq: ESC + “|N”
pPrinter->printText(ESC + _T("|N mPosWindows SDK Sample\r\n"), null); |
4 Check Status
Use the checkPrinterStatus function to check the current status of the printer.
//initilize mpos. … int status = pPrinter->checkPrinterStatus(); //finalize mpos. |
In the sample, the status value can be checked as a character string by adding the getPrinterStatusString function. Please refer to the B-gate SDK manual for definitions regarding the returned status values.
You can check the printer’s status in real time when using the asbEnable function and StatusUpdateEvent. Please refer to item 5-3-4-5 B-gate Demo - 2 Printer StatusUpdateEvent Registrationregistration’.
5 Pagemode
The Pagemode Sample is a sample that prints 1 image, 1 text, 1 1-D barcode, and 1 QRCode after turning them 90 degrees counter-clockwise. The cutPaper function has been added to cut the paper after printing the Pagemode contents.
The following procedure is required to use Pagemode.
1) Configure Pagemode and printing area
//initilize mpos. … pPrinter->setPagemode(MPOS_PRINTER_PAGEMODE_IN); // Enter pagemode pPrinter->setPagemodePrintArea(0, 0, 500, 600); //Configure printing area from (0,0) to (500,600) |
2) Configure printing direction and coordinates, add content to be printed (repeat)
pPrinter->setPagemodeDirection(MPOS_PRINTER_PD_RIGHT90); //Configure printing direction pPrinter->setPagemodePosition(100, 80); //Configure printing coordinates pPrinter->printBitmapFile(_T("Logo.bmp"), MPOS_IMAGE_WIDTH_ASIS, MPOS_ALIGNMENT_CENTER, 0, FALSE, FALSE); //Add image print at coordinates right above pPrinter->setPagemodePosition(100, 210); // Configure printing coordinates pPrinter->printText(ESC +_T("|bCpagemode test") + CRLF, NULL); //Add image print at coordinates right above pPrinter->setPagemodePosition(100, 350); // Configure printing coordinates pPrinter->print1DBarcode(_T("1234567890123456"), MPOS_BARCODE_CODE128, 60, 2, //At coordinates right above MPOS_ALIGNMENT_LEFT, MPOS_BARCODE_TEXTBELOW); //Add 1D barcode pPrinter->setPagemodePosition(330, 130); // Configure printing coordinates pPrinter->printQRCode(_T("http://www.bixolon.com"), MPOS_BARCODE_QRCODE_MODEL1, // At coordinates right above MPOS_ALIGNMENT_CENTER, 6, MPOS_QRCODE_ECC_LEVEL_H);//Add QRCode |
Only call the printing direction configuration once when you wish to change the direction.
3) Cancel after printing Pagemode
pPrinter->setPagemode(MPOS_PRINTER_PAGEMODE_OUT); //Cancel after printing pagemode pPrinter->cutPaper(MPOS_PRINTER_FEEDCUT); //Cut after feeding //finalize mpos. |
Label Print Sample
This is a sample on how to use a label printer. The printed label is made up of Vector Font, a barcode, and lines.
1 Label Printer Sample
The Label Printer Sample is made up of printed text/barcode/image file.
//initilize mpos. … pLabelPrinter->setLength(1200, 0, MPOS_LABEL_MEDIA_GAP, 0); //Configure label paper pLabelPrinter->drawBlock(40, 20, 265, 420, MPOS_LABEL_DRAW_BLOCK_OPTION_BOX, 3); pLabelPrinter->drawBlock(164, 20, 166, 420, MPOS_LABEL_DRAW_BLOCK_OPTION_LINE_OVERWRITING, 1);//li pLabelPrinter->drawTextVectorFont(_T("mPos Windows"), 50, 400, MPOS_LABEL_VECTOR_FONT_ASCII, 30, 30, 0, MPOS_LABEL_ROTATION_DEGREES_270, FALSE, FALSE, FALSE, FALSE, MPOS_LABEL_ALIGNMENT_LEFT); pLabelPrinter->drawTextVectorFont(_T("Label Sample"), 90, 400, MPOS_LABEL_VECTOR_FONT_ASCII, 30, 30, 0, MPOS_LABEL_ROTATION_DEGREES_270, FALSE, FALSE, FALSE, FALSE, MPOS_LABEL_ALIGNMENT_LEFT); pLabelPrinter->drawBarcodeQRCode(_T("http://bixolon.com"), 50, 150, 4, MPOS_LABEL_BARCODE_QRCODE_MODEL_1, MPOS_LABEL_BARCODE_QRCODE_ECC7, MPOS_LABEL_ROTATION_DEGREES_270); pLabelPrinter->drawBarcode1D(_T("1234567890123456"), 170, 400, MPOS_LABEL_BARCODE_TYPE_CODE128, 3, 2, 60, 3, 0, MPOS_LABEL_ROTATION_DEGREES_270); pLabelPrinter->printBuffer(1); //Print 1 copy of contents saved in label buffer //finalize mpos. |
* The printBuffer function must be called for the label printer to print contents saved in the buffer.
pLabelPrinter->printBuffer(1); //Print 1 copy of contents saved in label buffer |
2 drawImageFile
This prints image files. It prints the “Logo.bmp” file at the coordinates (100,100).
pLabelPrinter->drawImageFile(_T("Logo.bmp"), 100, 100, MPOS_IMAGE_WIDTH_ASIS); |
3 drawBlock
This prints lines or boxes. It can draw a range of lines depending on the 5th parameter, which is the ‘option’ value. Please refer to the B-gate SDK manual for more details.
pLabelPrinter->drawBlock(40, 20, 265, 420, MPOS_LABEL_DRAW_BLOCK_OPTION_BOX, 3); |
4 drawTextDeviceFont
This prints character strings as the Device font. Differently from the Vector font, the Device font’s size is predetermined and can only be adjusted by its scaling unit.
pLabelPrinter->drawTextDeviceFont(_T("mPos Windows"), 350, 400, MPOS_LABEL_DEVICE_FONT_10PT, 2, 2, 0, MPOS_LABEL_ROTATION_DEGREES_270, FALSE, FALSE, FALSE, MPOS_LABEL_ALIGNMENT_LEFT); |
The label printer’s configurations can be changed through functions.
5 setLength
You can select the length and type of label paper.
The label printer may malfunction if the paper type is configured differently.
pLabelPrinter->setLength(1200, 0, MPOS_LABEL_MEDIA_GAP, 0); //Gap paper //or pLabelPrinter->setLength(1200, 0, MPOS_LABEL_MEDIA_BLACKMARK, 0); //Black mark paper //or pLabelPrinter->setLength(1200, 0, MPOS_LABEL_MEDIA_CONTINUEOUS, 0); //Continuous paper |
6 setWidth
This configures the label paper width. The standard position (0, 0) may change depending on the configuration values.
pLabelPrinter->setWidth(834); |
7 setMargin
This configures the left and top margins. The standard position (0, 0) may change depending on the configuration values.
pLabelPrinter->setMargin(50, 100); |
8 setPrintingType
This configures the Direct Thermal method and the Thermal Transfer method.
pLabelPrinter->setPrintingType(MPOS_LABEL_PRINTING_TYPE_DIRECT_THERMAL); //or pLabelPrinter->setPrintingType(MPOS_LABEL_PRINTING_TYPE_THERMAL_TRANSFER); |
9 Other Configurations
You can configure the printing speed, density, and direction.
//Printing speed pLabelPrinter->setSpeed(4); //Printing density pLabelPrinter->setDensity(10); //Printing direction pLabelPrinter->setOrientation(MPOS_LABEL_PRINTING_DIRECTION_TOP_TO_BOTTOM); //or pLabelPrinter->setOrientation(MPOS_LABEL_PRINTING_DIRECTION_BOTTOM_TO_TOP); |
Input Device Sample
This sample shows how to use an input device.
Input devices include MSR, Barcode Scanner, DallasKey, RFID, NFC, HID, Scale, and USB to Serial devices, and MSR, Barcode Scanner, DallasKey, RFID, NFC devices can be used in the sample. Configure the appropriate interface in the B-gate you wish to connect and select the device you wish to use.
For example, in order to use a Scanner device, click on the ‘Scanner Connect’ button and then read a barcode with the Barcode Scanner connected to the B-gate. The barcode data will then appear in the text box at the bottom.
1 Input Device DataEvent Sample
The Input Device DataEvent Sample is a sample of how to read an input device’s data.
//initilize barcode scanner device. … //data event registration pScanner->setDataEvent(CInputDeviceDlg::DataEvent); //Once a device is closed, DataEvent cannot be received. |
The following is a sample that processes DataEvent. The inputted data length and data is printed in the text box.
void __stdcall CInputDeviceDlg::DataEvent(LPCTSTR data) { CString str = _T(""); str.Format(_T("Data length=%d\n%s\n"), _tcslen(data), data); pDlg->SetDlgItemText(IDC_STATIC_DATA, str); } |
B-gate Demo
This is made up of the printer, Customer Display, input device (Barcode Scanner) and Cashdrawer device.
Selecting the ‘Printer Receipt’ button will connect the printer, Customer Display, and the Barcode Scanner in that order. If the connection is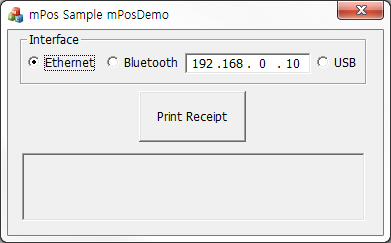
1 Character String Related Customer Display
This is a description of the function that displays and deletes character strings in the Customer Display.
1) displayString
This prints character strings on the Customer Display screen.
pMPosBCD->displayString(_T("Subtotal 895.00Total 1,000.00")); |
2) clearScreen
This clears the entire Customer Display screen.
pMPosBCD->clearScreen(); |
2 Printer StatusUpdateEvent Registration
You can check a printer’s status in real time when using the asbEnable function and StatusUpdateEvent.
pMPosPrinter->asbEnable(TRUE); //asb enable. |
void __stdcall CmPosDemoDlg::StatusUpdateEvent(int nStatus) { CString str; str.Format(_T("%s Status Update\n"), getPrinterStatusString(nStatus)); pDlg->SetDlgItemText(IDC_STATIC_DATA, str); } |
3 Cashdrawer Open
pMPosPrinter->openDrawer(MPOS_CASHDRAWER_PIN2); |